Predict#
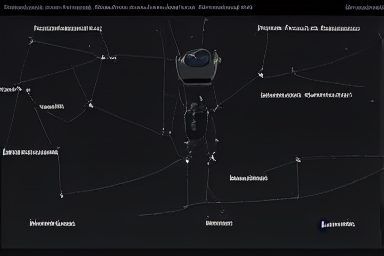
Make predictions on any model in a project or deployment.
Usage#
A core tenet of drx
is to make predictions easy across all problem types (multilabel, timeseries regression,
clustering, etc.)
Making predictions with drx
is done for any model by invoking the predict
or
predict_proba
methods and passing in a dataframe.
See also
The below examples assume you have already trained or deployed a model. To run the examples below, first follow the train and deploy sections of the AutoML guide.
Predict with a model#
test_df = pd.read_csv('https://s3.amazonaws.com/datarobot_public_datasets/10K_2007_to_2011_Lending_Club_Loans_v2_mod_20.csv')
predictions = model.predict(test_df)
class_probs = model.predict_proba(test_df)
Predict with a deployment#
drx
uses the same syntax for making predictions with a deployment as it does for a model. With deployments,
users may also optionally request prediction explanations or run predictions in batch mode.
predictions = deployment.predict(test_df)
predictions_with_explanations = deployment.predict(test_df, max_explanations=5)
batch_predictions = deployment.predict(test_df, batch_mode=True)
Clustering and multilabel
Note that DataRobot does not support prediction explanations for clustering and multilabel deployments.
Reshape prediction explanations#
drx
comes with functions for reshaping prediction explanations. These utilities
can be useful in visualizing explanations or incorporating them into downstream workflows, see
feature explanation clustering as an example.
# Melt predictions to longform
melted_preds = drx.melt_explanations(predictions_with_explanations)
# Featurize explanations
featurized_explanations = drx.featurize_explanations(predictions_with_explanations)
print(melted_preds)
print(featurized_explanations)
row_id |
explanation_number |
feature_name |
actual_value |
strength |
qualitative_strength |
|
---|---|---|---|---|---|---|
0 |
0 |
1 |
inq_last_6mths |
3.0 |
0.289366 |
+++ |
1 |
0 |
2 |
pub_rec |
1.0 |
0.276196 |
+++ |
2 |
0 |
3 |
purpose |
credit_card |
-0.236146 |
– |
3 |
0 |
4 |
verification_status |
VERIFIED - income |
0.177281 |
++ |
4 |
0 |
5 |
addr_state |
TX |
-0.114717 |
– |
5 |
1 |
1 |
inq_last_6mths |
-1.70278 |
— |
|
6 |
1 |
2 |
revol_util |
-0.834772 |
— |
|
7 |
1 |
3 |
emp_title |
0.293294 |
++ |
|
8 |
1 |
4 |
verification_status |
not verified |
-0.12343 |
– |
addr_state |
annual_inc |
earliest_cr_line (Month) |
emp_title |
inq_last_6mths |
pub_rec |
purpose |
revol_util |
title |
verification_status |
zip_code |
|
---|---|---|---|---|---|---|---|---|---|---|---|
0 |
-0.114717 |
0 |
0 |
0 |
0.289366 |
0.276196 |
-0.236146 |
0 |
0 |
0.177281 |
0 |
1 |
0 |
0 |
-0.063429 |
0.293294 |
-1.70278 |
0 |
0 |
-0.834772 |
0 |
-0.12343 |
0 |
2 |
0 |
-0.319414 |
0.0782486 |
0 |
0 |
0 |
0 |
-0.412047 |
0.0909408 |
0.177281 |
0 |
3 |
0 |
0.296704 |
0 |
0.293294 |
-0.503236 |
0 |
0.436913 |
-0.487348 |
0 |
0 |
0 |
4 |
0.144655 |
0 |
0 |
0 |
-0.177957 |
0 |
-0.1361 |
-0.577191 |
0 |
0 |
-0.106319 |
Predict using secondary models#
By default, drx uses the top model on the DataRobot leaderboard to make predictions. Users can interact
with other models in a project using the drx.Model.from_url()
method.
Predict#
secondary_model = drx.Model.from_url("https://app.datarobot.com/projects/project_id_here/models/model_id_here/")
secondary_model.predict(scoring_data_here)
Deploy#
deployment = secondary_model.deploy()
API Reference#
|
AutoML orchestrator. |
|
DataRobot training model. |
|
DataRobot ML Ops deployment. |
|
Deploy a model to MLOps. |
|
Melt Dataframe of explanations into long form columns. |
Featurizes a dataframe of explanations into strength values by feature. |